Proxy Azure OpenAI Requests
Azure OpenAI Service is a fully managed service that provides unified REST API access to OpenAI's language models, such as GPT-4 and GPT-3.5-Turbo. They can be easily integrated into applications to add capabilities such as content generation, text completion, semantic search, and more.
This guide will walk you through the process of integrating APISIX with Azure OpenAI Service.
Prerequisite(s)
- Have an Azure account and log into the Azure portal.
- Install Docker.
- Install cURL to send requests to the services for validation.
- Follow the Getting Started Tutorial to start a new APISIX instance in Docker or on Kubernetes.
Request Access to Azure OpenAI Service
Before proceeding, you should first request access to Azure OpenAI Service as part of Microsoft's commitment to responsible AI by filling out a registration form.
Please request the access for GPT-3.5, GPT-3.5 Turbo, GPT-4, GPT-4 Turbo, and/or Embeddings Models to follow along.
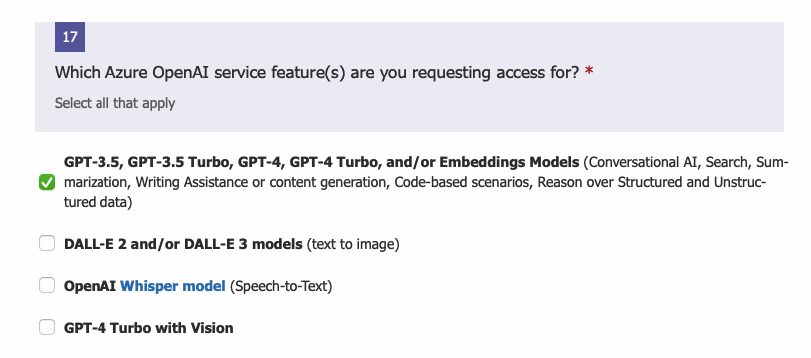
Deploy an Azure OpenAI Service
Once the access is granted, search for Azure AI services, navigate to the Azure OpenAI in the left panel, and click Create Azure OpenAI:
Fill out the project and instance details:
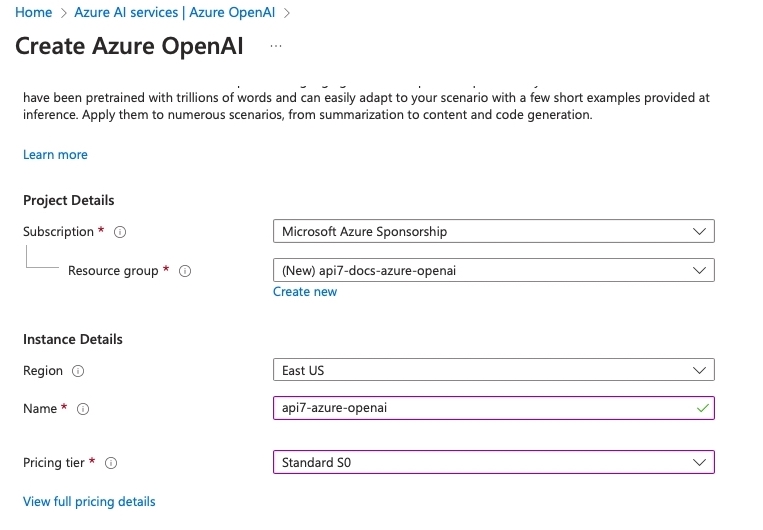
In the Network tab, select the All networks option, or adjust accordingly per your infrastructure:
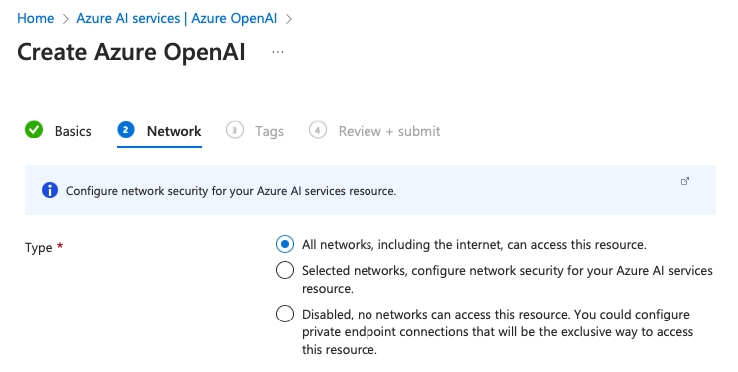
Continue with the setup until the deployment is complete:
Obtain API Information
Go to the Azure AI Foundry and select Chat. Click View Code:
Switch the command to curl and select the key authentication tab:
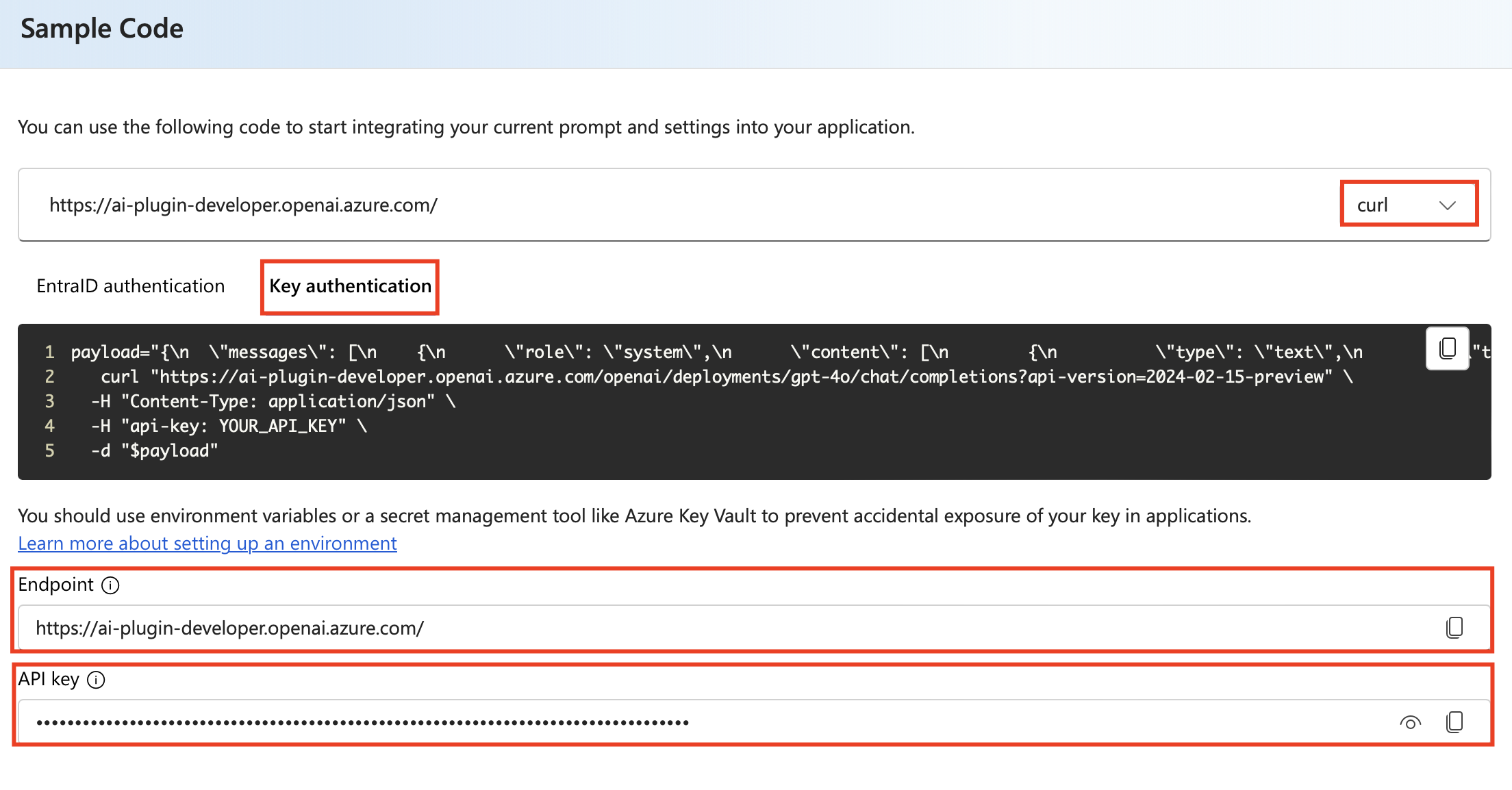
Copy the generated curl command, endpoint, and API key.
You can optionally save your API key to an environment variable:
export AZ_OPENAI_API_KEY=57cha9ee8e8a89a12c0aha174f180f4 # replace with your API key
Create a Route to Azure OpenAI
There are two main approaches to configure this route:
- Create a route to the Azure OpenAI endpoint and attach API key using
proxy-rewrite
. - Create a route with the
ai-proxy
plugin and override the default endpoint with the Azure OpenAI endpoint.
Using proxy-rewrite
Create a route to the Azure API endpoint and use proxy-rewrite
plugin to attach the API key to request headers:
- Admin API
- ADC
- Ingress Controller
curl "http://127.0.0.1:9180/apisix/admin/routes" -X PUT -d '{
"id": "azure-openai-route",
"uri": "/openai/deployments/*",
"plugins": {
"proxy-rewrite": {
"headers": {
"set": {
"api-key": "'"$AZ_OPENAI_API_KEY"'"
}
}
}
},
"upstream": {
"scheme": "https",
"pass_host": "node",
"nodes": {
"ai-plugin-developer.openai.azure.com:443": 1
},
"type": "roundrobin"
}
}'
❶ Configure the route to Azure API's chat endpoint.
❷ Attach the API key to the request in the api-key
header.
❸ Set the scheme to HTTPS.
❹ Set the upstream node to Azure's API domain and the listening port to 443
.
services:
- name: Azure OpenAI Service
routes:
- uris:
- /openai/deployments/*
name: azure-openai-route
plugins:
proxy-rewrite:
headers:
set:
api-key: 57cha9ee8e8a89a12c0aha174f180f4
upstream:
scheme: https
pass_host: node
nodes:
- host: ai-plugin-developer.openai.azure.com
port: 443
weight: 1
type: roundrobin
❶ Configure the route to Azure API's chat endpoint.
❷ Attach the API key to the request in the api-key
header.
❸ Set the scheme to HTTPS.
❹ Set the upstream node to Azure's API domain and the listening port to 443
.
Synchronize the configuration to APISIX:
adc sync -f adc.yaml
Create a Kubernetes manifest file to configure a route using the ApisixRoute custom resource:
apiVersion: apisix.apache.org/v2
kind: ApisixRoute
metadata:
name: azure-openai-route
namespace: ingress-apisix
spec:
http:
- name: azure-openai-route
match:
paths:
- /openai/deployments/*
upstreams:
- name: azure-openai-upstream
plugins:
- name: proxy-rewrite
enable: true
config:
headers:
api-key: 57cha9ee8e8a89a12c0aha174f180f4
❶ Configure the route to Azure API's chat endpoint.
❷ Attach the API key to the request in the api-key
header. Alternatively, you can use Kubernetes secrets, Helm values file, or kustomize to handle environment variables.
Create another Kubernetes manifest file to configure how the upstream should pass the host header using the ApisixUpstream custom resource:
apiVersion: apisix.apache.org/v2
kind: ApisixUpstream
metadata:
name: azure-openai-upstream
namespace: ingress-apisix
spec:
scheme: https
passHost: node
loadbalancer:
type: roundrobin
externalNodes:
- type: Domain
name: ai-plugin-developer.openai.azure.com
port: 443
❶ Set the upstream scheme to HTTPS.
❷ Set the upstream node to Azure's API domain and the listening port to 443
.
Apply the configurations to your cluster:
kubectl apply -f azure-openai-route.yaml -f azure-openai-upstream.yaml
Using ai-proxy
Create a route and configure the ai-proxy
plugin as such:
- Admin API
- ADC
- Ingress Controller
curl "http://127.0.0.1:9180/apisix/admin/routes" -X PUT \
-H "X-API-KEY: ${ADMIN_API_KEY}" \
-d '{
"id": "ai-proxy-route",
"uri": "/anything",
"methods": ["POST"],
"plugins": {
"ai-proxy": {
"provider": "openai-compatible",
"auth": {
"header": {
"api-key": "'"$AZ_OPENAI_API_KEY"'"
}
},
"model": "gpt-4",
},
"override": {
"endpoint": "https://api7-auzre-openai.openai.azure.com/openai/deployments/gpt-4/chat/completions?api-version=2024-02-15-preview"
}
}
}
}'
❶ Set the provider to openai-compatible
when integrating with OpenAI-compatible API with a custom endpoint.
❷ Attach Azure OpenAI API key in the header.
❸ Override with the Azure OpenAI endpoint.
services:
- name: Azure OpenAI Service
routes:
- uris:
- /anything
name: azure-openai-route
plugins:
ai-proxy:
provider: openai-compatible
auth:
header:
api-key: 57cha9ee8e8a89a12c0aha174f180f4
model: gpt-4
override:
endpoint: https://api7-auzre-openai.openai.azure.com/openai/deployments/gpt-4/chat/completions?api-version=2024-02-15-preview
❶ Set the provider to openai-compatible
when integrating with OpenAI-compatible API with a custom endpoint.
❷ Attach Azure OpenAI API key in the header.
❸ Override with the Azure OpenAI endpoint.
Synchronize the configuration to APISIX:
adc sync -f adc.yaml
Create a Kubernetes manifest file to configure a route using the ApisixRoute custom resource:
apiVersion: apisix.apache.org/v2
kind: ApisixRoute
metadata:
name: azure-openai-route
namespace: ingress-apisix
spec:
http:
- name: azure-openai-route
match:
paths:
- /anything
plugins:
- name: ai-proxy
enable: true
config:
provider: openai-compatible
auth:
header:
api-key: 57cha9ee8e8a89a12c0aha174f180f4
model: gpt-4
override:
endpoint: https://api7-auzre-openai.openai.azure.com/openai/deployments/gpt-4/chat/completions?api-version=2024-02-15-preview
❶ Set the provider to openai-compatible
when integrating with OpenAI-compatible API with a custom endpoint.
❷ Attach Azure OpenAI API key in the header.
❸ Override with the Azure OpenAI endpoint.
Apply the configuration to your cluster:
kubectl apply -f azure-openai-route.yaml
Verify
- Admin API
- ADC
- Ingress Controller
First, expose the service port to your local machine by port forwarding:
kubectl port-forward svc/apisix-gateway 9080:80 &
The command above runs in the background and maps port 80
of the apisix-gateway
service to port 9080
on the local machine.
If you have configured the route with proxy-rewrite
, send the following request to the route:
curl "http://127.0.0.1:9080/openai/deployments/gpt-4o/chat/completions?api-version=2024-02-15-preview" -X POST \
-H "Content-Type: application/json" \
-d '{
"messages": [
{
"role": "system",
"content": "You are an AI assistant that helps people find information."
},
{
"role": "user",
"content": "Write me a 50-word introduction for Apache APISIX."
}
]
}'
If you have configured the route with ai-proxy
, send the following request to the route:
curl "http://127.0.0.1:9080/anything" -X POST \
-H "Content-Type: application/json" \
-d '{
"messages": [
{
"role": "system",
"content": "You are an AI assistant that helps people find information."
},
{
"role": "user",
"content": "Write me a 50-word introduction for Apache APISIX."
}
]
}'
In both cases, you should receive a response similar to the following:
{
"choices": [
{
...,
"message": {
"content": "Apache APISIX is a modern, cloud-native API gateway built to handle high-performance and low-latency use cases. It offers a wide range of features, including load balancing, rate limiting, authentication, and dynamic routing, making it an ideal choice for microservices and cloud-native architectures.",
"role": "assistant"
}
}
],
...
}
Next Steps
You have now learned how to integrate APISIX with Azure OpenAI Service. See Azure OpenAI Service REST API reference to learn more.
If you would like to stream the Azure API response, you can set the stream
parameter to true
for Azure OpenAI Service and use the proxy-buffering
plugin in APISIX to disable NGINX's proxy_buffering
directive, which avoids the server-sent events (SSE) being buffered.
In addition, you can integrate more capabilities that APISIX offers, such as rate limiting and caching, to improve system availability and user experience.